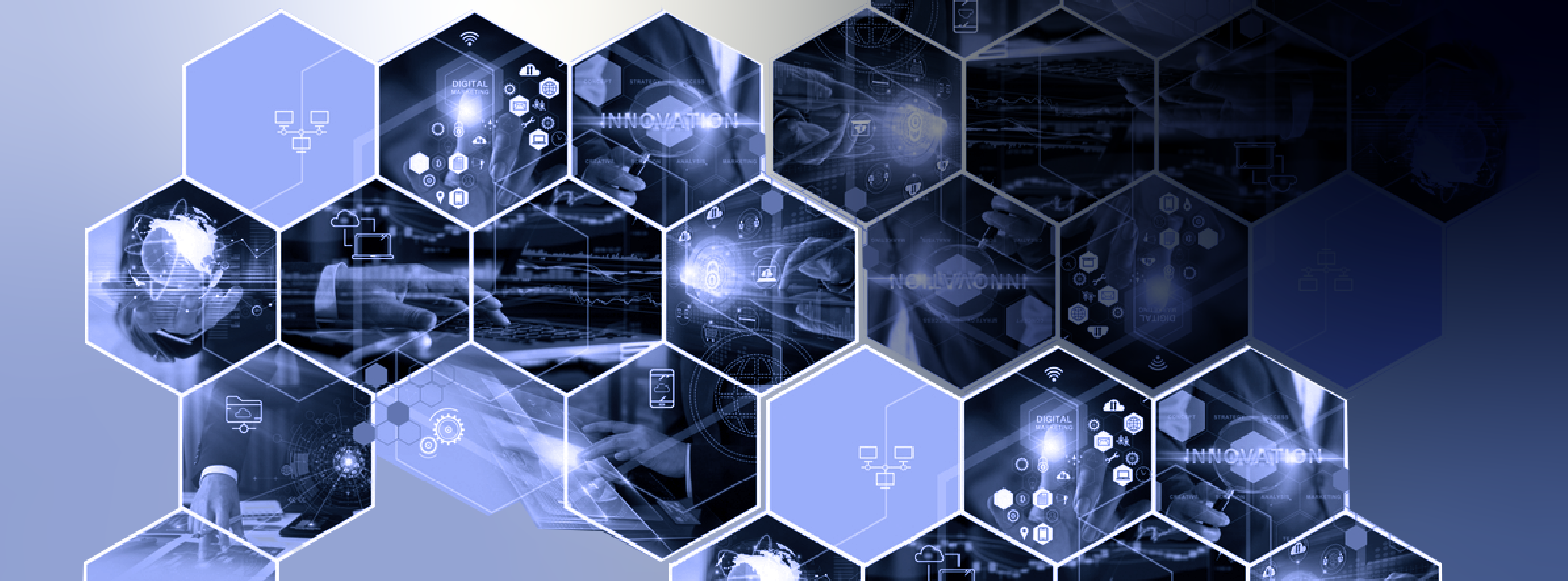
Ön Koşul
Eğitim Hakkında
Full Stack Spring & React Eğitiminin temel amacı; Spring ve React alanında nitelikli uzmanlar yetiştirmek ve sektörde çalışan, sektöre giriş yapmak isteyen veya kariyerini değiştirmeyi hedefleyen kişilerin kariyer planlamalarına yön vermektir.
Eğitim Programımızda 3 ana modül vardır;
- Modül - Yeni başlayan kişilere hitap eder, Java ve Programlama öğretilir.
- Modül - Spring Boot Service Mimarisi Uzmanı yetiştirilir.
- Modül - React ile Yazılım Uzmanı yetiştirilir.
Sertifika
Eğitimlerimize %80 oranında katılım gösterilmesi ve eğitim müfredatına göre uygulanacak sınav/projelerin başarıyla tamamlanması durumunda, eğitimin sonunda dijital ve QR kod destekli “BT Akademi Başarı Sertifikası” verilmektedir.
Eğitim İçeriği
Getting Started with Java
• How to Get Java
• A First Java Program
• About Your First Java Program
• Compiling and Interpreting Applications
• The JDK Directory Structure
IDE
• Objectives
• Introduction
• Installing
• Running for the First Time
• Editors, Views, and Perspectives
• Creating a Project and Class
• Running a Java Application
• Debugging a Java Application
• Importing Existing Java Code
Datatypes and Variables
• Objectives
• Primitive Datatypes
• Declarations
• Variable Names
• Numeric Literals
• Character Literals
• Strings and String Comparisons
• String API Documentation
• Immutable Strings
• String Literals
• Arrays
• More Arrays
• Non-Primitive Datatypes
• The Dot Operator
Operators and Expressions
• Assignment Operator
• Arithmetic Operators
• Relational Operators
• Logical Operators
• Increment and Decrement Operators
• Operate-Assign Operators
• The Conditional Operator
• Operator Precedence
• Implicit Type Conversions
• The Cast Operator
Control Flow
• Statements
• Conditional (if) Statements
• Adding an else if
• Conditional (switch) Statements
• while and do-while Loops
• for Loops
• Looping Through an Array
• Enhanced for Loop
• The continue Statement
• The break Statement
Methods
• Methods
• Calling Methods
• Defining Methods
• Method Parameters
• Scope
Object-Oriented Programming
• Introduction to Object-Oriented Programming
• Classes and Objects
• Fields and Methods
• Encapsulation
• Access Control
• Inheritance
• Polymorphism
• Demonstrating Polymorphism with Arrays
• Best Practices
Objects and Classes
• Objectives
• Defining a Class
• Creating an Object
• Viewing Object Creation in the Debugger
• Instance Data and Class Data
• Using the final and this Keywords
• Avoiding Code Duplication
• Defining Constructors
• Calling Constructors
• Access Modifiers
• Encapsulation
Using Java Objects
• StringBuilder and StringBuffer
• The toString() Method
• The equals() Method
• The hashCode() Method
• Parameter Passing
• Parameter Passing Example
• Enums
Inheritance in Java
• Inheritance
• Inheritance in Java
• Casting
• Method Overriding
• Polymorphism
• super
• The Object Class
Advanced Inheritance and Generics
• Abstract Classes
• Interfaces
• Using Interfaces
• Collections
• Generics
• Comparable
Exception Handling
• Catching Exceptions
• The finally Block
• Exception Methods
• Declaring Exceptions
• Defining and Throwing Exceptions
• Errors and RuntimeExceptions
Input/Output Streams
• Objectives
• Overview of Streams
• Bytes vs. Characters
• Converting Byte Streams to Character Streams
• The File Object
• Read a Binary File into a Byte Array
• Read a Binary File into a Byte Array (Continued)
• DataInputStream and DataOutputStream
• BufferedReader and PrintWriter
• Reading and Writing Objects
• Closing Streams
Collection Classes
• The Collections Framework
• The Set Interface
• Set Implementation Classes
• The List Interface
• List Implementation Classes - ArrayList
• List Implementation Classes - LinkedList
• The Queue Interface
• Queue Implementation Classes
• The Map Interface
• Map Implementation Classes
Spring Boot
• Spring Boot Overview
• Spring Projects
• Project Structure
• Spring Boot Project Files
• Spring Boot Starters
• Application Properties
Spring Core
• Defining Dependency Injection
• Constructor Injection
• Autowired
• Component Scanning
• Qualifiers
• Lazy Initialization
• Bean Scopes
• Bean Lifecycle Methods
• Java Config Bean
JPA / Hibernate
• Configuration
• Entity
• Database Table
• JPA Annotations
• Primary Keys
• Querying Objects
• JQL
• Projections
• Custom Query
• Performance
• Native Query
• Pagination and Sorting
• JPA Listener
REST API
• Rest Service Overview
• JSON Format
• Rest Controller
• RequestMapping
• ResponseStatus Annotation
• Requestparam
• Requestbody
• PathVariable
• Validation
• RestControllerAdvice
• REST Exception Handling
• REST Global Exception Handling
• JSON Jackson Data Binding
• REST API Design
• DTO
• REST DAO
• Define Service Layer
• Rest Cache
• Global Filter
• RestTemplate
REST API Security
• Basic Configuration
• SecurityFilterChain
• AuthenticationProvider
• UserDetailsService
• RequestMatchers
• Basic Authentication
• JWT
• Restrict URLs based on Roles
• PasswordEncoder
• BCrypt Encryption
REST API Documenting and Versioning
• Postman Using
• Swagger with a Spring REST API
• OpenAPI
• Parameter and Schema
• Versioning a REST API
Introduction
• Features
• New Features of PostgreSQL
• Multi Version Concurrency Control
• Write-Ahead Logging
• Architectural Overview
• Limits
PostgreSQL System Architecture
• Architectural Summary
• Shared Memory
• Statement Processing
• Utility Processes
• Disk Read Buffering
• Write Buffering
• Background Writer Cleaning Scan
• Commit & Checkpoint
• Data Directory Layout
• Installation Directory Layout
• Page Layout
Creating and Managing Databases
• Object Hierarchy
• Creating Databases
• Creating Schemas
• Schema Search Path
• Roles, Users & Groups
• Access Control
• Data Types
• Primary Keys and Foreign Keys
• Constraints
• Create Table
• Insert
• Update
• Delete
• Alter Table
• Drop Table
• Check Constraint
• Joins
• Group By
• Having
• Count
• Limit
• Between
Dictionary & Configuration
• Catalog Schema
• Views/tables
• Functions
• Parameters
• Access Control
• Connection Settings
• Security and Authentication
• Memory Settings
• Query Planner Settings
• Log Management
• Background Writer Settings
Database Monitoring
• Database Statistics
• The Statistics Collector
• Database Statistic Tables
• Operating System Process Monitoring
• Current Sessions and Locks
• Log Slow Running Queries
• Disk Usage
Introduction to HTML5 & Setting things up
• Introduction to HTML5
• Features of HTML5
• Benefits of using HTML5
• Where and how is HTML5 used?
• HTML5 editors
• Downloading Visual Studio Code
Structure HTML5
• HTML tags?
• Structure of an HTML5 file
• File and folder structure
• How to create an HTML5 file?
• Heading Tags in HTML
• HTML5 Quotation and Citation tags
• Commenting out code in HTML5
• HTML5 attributes
• How to style in HTML5
Exploring HTML5 tags
• What are anchor tags?
• What are image tags?
• What are paragraph tags?
• What are break tags?
• What are table tags?
• List Tags
• Video Tag
• Audio Tag
Building a Form from Scratch
• Form tags
• Input Types
• Input attributes (size, read-only, disabled)
• Additional input attributes (Min, max, multiple, and placeholder)
• Required Inputs
Block and Inline Elements
• Introduction
• Examining Block and Inline Elements
• HTML Project
Introduction to CSS3
• Introduction
• Syntax
• Uses
• Types
• Selectors & Colors
Basics Properties of CSS3
• Background
• Borders
• Outline
• Height and width
• Margin and padding
Typography Properties of CSS3
• Text alignment
• Text decoration
• Text transform
• Text spacing
• Font family
• How to use font awesome icons
Selectors Properties in Depth of CSS3
• Display
• Positions
• Z-index
• Overflow
• Float
• DOM Model
• Advanced Selectors
• Pseudo-classes
• Pseudo-Elements
• Opacity and important
• Border-radius
• Box Shadow
Responsive Design
• Grid System
• Flexbox
• Media Queries
TypeScript Basics & Basic Types
• Module Introduction
• Using Types
• TypeScript Types vs JavaScript Types
• Important: Type Casing
• Working with Numbers, Strings & Booleans
• Type Assignment & Type Inference
• Understanding Types
• Object Types
• Nested Objects & Types
• Arrays Types
• Working with Tuples
• Working with Enums
• The 'any' Type
• Union Types
• Literal Types
• Type Aliases / Custom Types
• Type Aliases & Object Types
• Core Types & Concepts
• Function Return Types & 'void'
• Functions as Types
• Function Types & Callbacks
• Functions & Types
• The 'unknown' Type
• The 'never' Type
• Wrap Up
Classes & Interfaces
• What are Classes?
• Creating a First Class
• Compiling to JavaScript
• Constructor Functions & The 'this' Keyword
• 'private' and 'public' Access Modifiers
• Shorthand Initialization
• 'readonly' Properties
• Class Basics
• Inheritance
• Overriding Properties & The 'protected' Modifier
• Getters & Setters
• Static Methods & Properties
• Abstract Classes
• Singletons & Private Constructors
• A First Interface
• Using Interfaces with Classes
• Why Interfaces?
• Readonly Interface Properties
• Extending Interfaces
• Interfaces as Function Types
• Optional Parameters & Properties
• Compiling Interfaces to JavaScript
Generics
• Built-in Generics & What are Generics?
• Creating a Generic Function
• Working with Constraints
• Another Generic Function
• The 'keyof' Constraint
• Generic Classes
• A First Summary
• Generic Utility Types
• Generic Types vs Union Types
React Overview
• Create Project with TypeScript
• Lifecycle
• Architectural
• Single Page
• Components
• Props
• Libs
Data Types
• string
• number
• array
• date
• type
• interface
• propTypes
TypeScript
• Types
• Arrays
• Enums
• Functions
• Casting
• Custom Types
• Interface
• Type
• Generics
• Null and Optional Type
• Advanced Object
Component Nesting
• Functional Component
• Create Component
• Component push Props
• Child Components
• Using Component
• Props Children
React Router Dom
• Installations
• Pages
• Route Management
• BrowserRouter
• Routes
• Route
• Page push data
• Path Variable
• Query String
• Send Object
• NavLink
• useNavigate
• useParams / useLocation
• useSearchParams
• error Page
React Features
• JSX
• Virtual DOM
• One-way Data Binding
• Performance
• Extension
• Conditional Statements in JSX
• CSS Using
• CDN Using
• Style
• Events
• React Lists
• List Keys
• Control
• Loop Display
• React Performance
• React Developer Tools
• SEO
React Form
• FormEvent
• onSubmit
• preventDefault
• errorMessage
• Data Validate
• Formik lib
• Yup lib
Hooks
• useState
• useEffect
• createContext
• useContext
• useRef
• useMemo
• useCallback
• useReducer
• useTransition
• REST API Using
• Axios
• Promise, Async, Await
• Global Axios Config
• Api Call
• Return Model Type
• Interface Models
• Login
• Auth
• Role Based Access Control
• Global Auth Control
Redux
• Installations
• Enum Type Call
• Actions
• Reducers
• Store
• StateType
• useSelector
• useDispatch
Neden Bu Eğitimi Almalısınız ?
.
Önemli Notlar
Program ücretlerine KDV dahil değildir